HTTP
Server in the ADAM-5510 Ethernet Controller Series
Since the ADAM-5510
PC-based Controller Series are now equipped with Ethernet, Advantech
introduces the HTTP Server function which enables web monitoring
& control. In order to better understand this function, we have
illustrated the system process in the diagram below. This diagram
shows the software architecture of HTTP Server function. The HTTP
Server is built in the ADAM-5510/TCP (or ADAM-5510E/TCP) Ethernet
Controller. Whenever users open the IE Browser to acquire data from
the ADAM-5510/TCP Controller through the Internet or Intranet, it
will call on the existing web pages to provide a monitoring and
control portal. All of the commands from the web page must be linked
via a CGI program to the C control program which handles the read/write
functions in the ADAM-5000 I/O modules.
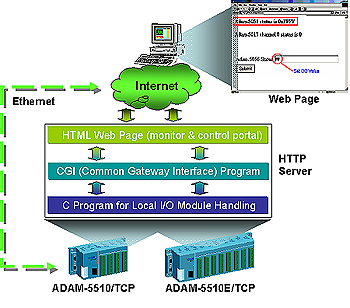
Basically, there
are three steps in the process of web monitoring & control:
1. Registration: Register an HTML name for the web page you designed
2. User login and invoke control program: After registration, users
can invoke local control program through the login server
3. Local I/O activated by local control program
The following
programming code is a quick example of data read from the ADAM-5000
I/O modules.
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <process.h>
#include "5510drv.h"
#include "CGI_Lib.h"
FILE *fp;
int number = 0;
int count = 1;
unsigned LocalDIO(void);
unsigned int LocalAIO(void);
void ReplaceStr(char *ptr_str1, char *ptr_str2, int len_str);
void main(void)
{
char * homepage_name = "index.htm";
Init501718(3);
if(!Http_Server_Reg(homepage_name))
// registration
return;
printf("Program
exiting...");
HttpDeRegister("index.htm");
}
int far Callback(HTTP_PARAMS far* psParams) // implement your program
in this function
{
static char *ptr_XX = 0;
static char *ptr_OO = 0;
char *tmpStr = 0;
static char Htm_Content[] = "HTTP/1.0 200 OK\r\n" // content
of html page, content=1
"Content-type: text/html\r\n\r\n" // means refreshes every
1 second
"<html><META HTTP-EQUIV=""Refresh""
content=1>"
"<b>Adam-5051 status is OOOOOOO</b><p>"
"<b>Adam-5017 channel 0 status is XXXXXXX</b>"
"</html>";
number++;
printf("Refresh %d times...\n", number);
if (!ptr_OO)
ptr_OO = strstr(Htm_Content, "OOO");
sprintf(tmpStr, "0x%X", LocalDIO());
ReplaceStr(ptr_OO, tmpStr, 7);
tmpStr = 0;
if (!ptr_XX)
ptr_XX = strstr(Htm_Content, "XXX");
sprintf(tmpStr, "%d", LocalAIO());
ReplaceStr(ptr_XX, tmpStr, 7);
HttpSendData(psParams->iHandle, Htm_Content, strlen(Htm_Content));
return RET_DONE;
}
unsigned LocalDIO(void) // set Adam-5056&5068 and return Adam-5051
Status
{
unsigned div, dov;
char dov1;
if(count%2==0)
{
dov = 0xffff;
dov1 = 0x0;
}
else
{
dov = 0x0000;
dov1 = 0xff;
}
count++;
if(count>100)
count = 1;
Set5068(&dov1,2,0,AByte); //slot 2
Set5056(&dov,1,0,AWord); //slot 1
Get5051(0,0,AWord,&div);
//slot 0
return ~div;
}
unsigned int
LocalAIO(void) //return Adam-5017 channel 0 status
{
unsigned int
aiv;
int ch, tmpcnt;
tmpcnt=0;
while(1)
{
if(AiUpdate(3,0)==0)
{
tmpcnt++;
Get501718(3, 0, &aiv);
if(tmpcnt>=8)
break;
}
}
return aiv;
}
void ReplaceStr(char
*ptr_str1, char *ptr_str2, int len_str) // replace string
{
int i;
for(i=0; i<len_str; i++)
ptr_str1[i] = 32;
for(i=0; i<strlen(ptr_str2); i++)
ptr_str1[i] = ptr_str2[i];
}
By implementing
the above programming code, users can read the following ADAM-5051/5017
data from the web browser.
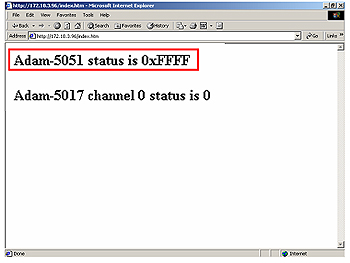
|